Note
Click here to download the full example code
Deploy Single Shot Multibox Detector(SSD) model¶
Author: Yao Wang Leyuan Wang
This article is an introductory tutorial to deploy SSD models with TVM. We will use GluonCV pre-trained SSD model and convert it to Relay IR
import tvm
from tvm import te
from matplotlib import pyplot as plt
from tvm import relay
from tvm.contrib import graph_executor
from tvm.contrib.download import download_testdata
from gluoncv import model_zoo, data, utils
/usr/local/lib/python3.7/dist-packages/gluoncv/__init__.py:40: UserWarning: Both `mxnet==1.6.0` and `torch==1.11.0+cpu` are installed. You might encounter increased GPU memory footprint if both framework are used at the same time.
warnings.warn(f'Both `mxnet=={mx.__version__}` and `torch=={torch.__version__}` are installed. '
Preliminary and Set parameters¶
Note
We support compiling SSD on both CPUs and GPUs now.
To get best inference performance on CPU, change target argument according to your device and follow the Auto-tuning a Convolutional Network for x86 CPU to tune x86 CPU and Auto-tuning a Convolutional Network for ARM CPU for arm CPU.
To get best inference performance on Intel graphics,
change target argument to opencl -device=intel_graphics
.
But when using Intel graphics on Mac, target needs to
be set to opencl only for the reason that Intel subgroup
extension is not supported on Mac.
To get best inference performance on CUDA-based GPUs,
change the target argument to cuda
; and for
OPENCL-based GPUs, change target argument to
opencl
followed by device argument according
to your device.
supported_model = [
"ssd_512_resnet50_v1_voc",
"ssd_512_resnet50_v1_coco",
"ssd_512_resnet101_v2_voc",
"ssd_512_mobilenet1.0_voc",
"ssd_512_mobilenet1.0_coco",
"ssd_300_vgg16_atrous_voc" "ssd_512_vgg16_atrous_coco",
]
model_name = supported_model[0]
dshape = (1, 3, 512, 512)
Download and pre-process demo image
Convert and compile model for CPU.
block = model_zoo.get_model(model_name, pretrained=True)
def build(target):
mod, params = relay.frontend.from_mxnet(block, {"data": dshape})
with tvm.transform.PassContext(opt_level=3):
lib = relay.build(mod, target, params=params)
return lib
/usr/local/lib/python3.7/dist-packages/mxnet/gluon/block.py:1389: UserWarning: Cannot decide type for the following arguments. Consider providing them as input:
data: None
input_sym_arg_type = in_param.infer_type()[0]
Downloading /workspace/.mxnet/models/ssd_512_resnet50_v1_voc-9c8b225a.zip from https://apache-mxnet.s3-accelerate.dualstack.amazonaws.com/gluon/models/ssd_512_resnet50_v1_voc-9c8b225a.zip...
0%| | 0/132723 [00:00<?, ?KB/s]
4%|4 | 5796/132723 [00:00<00:02, 57954.25KB/s]
10%|9 | 13230/132723 [00:00<00:01, 67590.58KB/s]
16%|#5 | 20648/132723 [00:00<00:01, 70584.15KB/s]
21%|##1 | 28077/132723 [00:00<00:01, 72045.15KB/s]
27%|##6 | 35481/132723 [00:00<00:01, 72760.20KB/s]
32%|###2 | 42965/132723 [00:00<00:01, 73457.99KB/s]
38%|###8 | 50506/132723 [00:00<00:01, 74090.70KB/s]
44%|####3 | 58051/132723 [00:00<00:01, 74522.03KB/s]
49%|####9 | 65629/132723 [00:00<00:00, 74913.14KB/s]
55%|#####5 | 73211/132723 [00:01<00:00, 75190.22KB/s]
61%|###### | 80781/132723 [00:01<00:00, 75345.48KB/s]
67%|######6 | 88481/132723 [00:01<00:00, 75846.26KB/s]
73%|#######2 | 96231/132723 [00:01<00:00, 76343.41KB/s]
78%|#######8 | 103917/132723 [00:01<00:00, 76497.47KB/s]
84%|########4 | 111632/132723 [00:01<00:00, 76684.31KB/s]
90%|########9 | 119321/132723 [00:01<00:00, 76742.76KB/s]
96%|#########5| 127081/132723 [00:01<00:00, 76997.16KB/s]
100%|##########| 132723/132723 [00:01<00:00, 74854.04KB/s]
Create TVM runtime and do inference .. note:
Use target = "cuda -libs" to enable thrust based sort, if you
enabled thrust during cmake by -DUSE_THRUST=ON.
def run(lib, dev):
# Build TVM runtime
m = graph_executor.GraphModule(lib["default"](dev))
tvm_input = tvm.nd.array(x.asnumpy(), device=dev)
m.set_input("data", tvm_input)
# execute
m.run()
# get outputs
class_IDs, scores, bounding_boxs = m.get_output(0), m.get_output(1), m.get_output(2)
return class_IDs, scores, bounding_boxs
for target in ["llvm", "cuda"]:
dev = tvm.device(target, 0)
if dev.exist:
lib = build(target)
class_IDs, scores, bounding_boxs = run(lib, dev)
Display result
ax = utils.viz.plot_bbox(
img,
bounding_boxs.numpy()[0],
scores.numpy()[0],
class_IDs.numpy()[0],
class_names=block.classes,
)
plt.show()
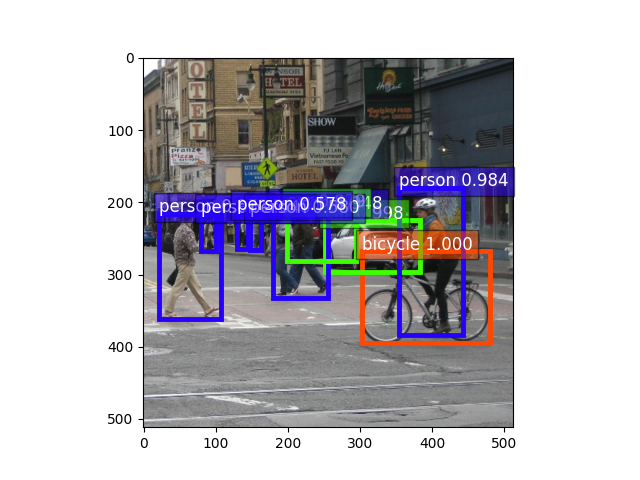
Total running time of the script: ( 2 minutes 32.530 seconds)